Reading in a From a File Java
This page discusses the details of reading, writing, creating, and opening files. There are a wide array of file I/O methods to choose from. To aid brand sense of the API, the post-obit diagram arranges the file I/O methods by complication.
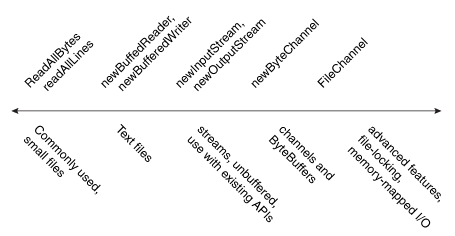
On the far left of the diagram are the utility methods readAllBytes
, readAllLines
, and the write
methods, designed for simple, common cases. To the right of those are the methods used to iterate over a stream or lines of text, such every bit newBufferedReader
, newBufferedWriter
, then newInputStream
and newOutputStream
. These methods are interoperable with the coffee.io
package. To the right of those are the methods for dealing with ByteChannels
, SeekableByteChannels
, and ByteBuffers
, such equally the newByteChannel
method. Finally, on the far correct are the methods that use FileChannel
for advanced applications needing file locking or memory-mapped I/O.
Note: The methods for creating a new file enable you to specify an optional prepare of initial attributes for the file. For example, on a file organisation that supports the POSIX set of standards (such as UNIX), you can specify a file owner, group owner, or file permissions at the fourth dimension the file is created. The Managing Metadata page explains file attributes, and how to access and ready them.
This page has the following topics:
- The
OpenOptions
Parameter - Ordinarily Used Methods for Small Files
- Buffered I/O Methods for Text Files
- Methods for Unbuffered Streams and Interoperable with
coffee.io
APIs - Methods for Channels and
ByteBuffers
- Methods for Creating Regular and Temporary Files
The OpenOptions
Parameter
Several of the methods in this department take an optional OpenOptions
parameter. This parameter is optional and the API tells you what the default beliefs is for the method when none is specified.
The following StandardOpenOptions
enums are supported:
-
WRITE
– Opens the file for write access. -
Suspend
– Appends the new data to the end of the file. This option is used with theWRITE
orCREATE
options. -
TRUNCATE_EXISTING
– Truncates the file to zippo bytes. This option is used with theWRITE
option. -
CREATE_NEW
– Creates a new file and throws an exception if the file already exists. -
CREATE
– Opens the file if it exists or creates a new file if it does not. -
DELETE_ON_CLOSE
– Deletes the file when the stream is airtight. This choice is useful for temporary files. -
Sparse
– Hints that a newly created file will be thin. This advanced choice is honored on some file systems, such every bit NTFS, where large files with information "gaps" can be stored in a more than efficient way where those empty gaps do not consume disk space. -
SYNC
– Keeps the file (both content and metadata) synchronized with the underlying storage device. -
DSYNC
– Keeps the file content synchronized with the underlying storage device.
Commonly Used Methods for Small-scale Files
Reading All Bytes or Lines from a File
If yous accept a small-ish file and you would like to read its entire contents in one pass, yous tin use the readAllBytes(Path)
or readAllLines(Path, Charset)
method. These methods take intendance of most of the work for you lot, such as opening and closing the stream, but are not intended for handling large files. The following code shows how to employ the readAllBytes
method:
Path file = ...; byte[] fileArray; fileArray = Files.readAllBytes(file);
Writing All Bytes or Lines to a File
Y'all can use one of the write methods to write bytes, or lines, to a file.
-
write(Path, byte[], OpenOption...)
-
write(Path, Iterable< extends CharSequence>, Charset, OpenOption...)
The post-obit code snippet shows how to use a write
method.
Path file = ...; byte[] buf = ...; Files.write(file, buf);
Buffered I/O Methods for Text Files
The coffee.nio.file
bundle supports aqueduct I/O, which moves data in buffers, bypassing some of the layers that can bottleneck stream I/O.
Reading a File past Using Buffered Stream I/O
The newBufferedReader(Path, Charset)
method opens a file for reading, returning a BufferedReader
that can be used to read text from a file in an efficient way.
The following code snippet shows how to use the newBufferedReader
method to read from a file. The file is encoded in "The states-ASCII."
Charset charset = Charset.forName("Usa-ASCII"); try (BufferedReader reader = Files.newBufferedReader(file, charset)) { String line = null; while ((line = reader.readLine()) != aught) { System.out.println(line); } } catch (IOException x) { Arrangement.err.format("IOException: %due south%n", x); }
Writing a File past Using Buffered Stream I/O
You tin can use the newBufferedWriter(Path, Charset, OpenOption...)
method to write to a file using a BufferedWriter
.
The post-obit lawmaking snippet shows how to create a file encoded in "U.s.-ASCII" using this method:
Charset charset = Charset.forName("US-ASCII"); Cord s = ...; try (BufferedWriter writer = Files.newBufferedWriter(file, charset)) { writer.write(s, 0, s.length()); } catch (IOException x) { Arrangement.err.format("IOException: %s%north", x); }
Methods for Unbuffered Streams and Interoperable with java.io
APIs
Reading a File by Using Stream I/O
To open up a file for reading, you can utilize the newInputStream(Path, OpenOption...)
method. This method returns an unbuffered input stream for reading bytes from the file.
Path file = ...; try (InputStream in = Files.newInputStream(file); BufferedReader reader = new BufferedReader(new InputStreamReader(in))) { Cord line = zilch; while ((line = reader.readLine()) != null) { Arrangement.out.println(line); } } catch (IOException x) { Organisation.err.println(ten); }
Creating and Writing a File by Using Stream I/O
You can create a file, append to a file, or write to a file past using the newOutputStream(Path, OpenOption...)
method. This method opens or creates a file for writing bytes and returns an unbuffered output stream.
The method takes an optional OpenOption
parameter. If no open options are specified, and the file does non be, a new file is created. If the file exists, it is truncated. This option is equivalent to invoking the method with the CREATE
and TRUNCATE_EXISTING
options.
The following case opens a log file. If the file does non exist, it is created. If the file exists, it is opened for appending.
import static java.nio.file.StandardOpenOption.*; import java.nio.file.*; import coffee.io.*; public form LogFileTest { public static void chief(String[] args) { // Catechumen the string to a // byte array. Cord south = "Hello World! "; byte information[] = s.getBytes(); Path p = Paths.get("./logfile.txt"); try (OutputStream out = new BufferedOutputStream( Files.newOutputStream(p, CREATE, Suspend))) { out.write(data, 0, data.length); } catch (IOException x) { System.err.println(ten); } } }
Methods for Channels and ByteBuffers
Reading and Writing Files by Using Aqueduct I/O
While stream I/O reads a character at a time, channel I/O reads a buffer at a time. The ByteChannel
interface provides basic read
and write
functionality. A SeekableByteChannel
is a ByteChannel
that has the adequacy to maintain a position in the channel and to change that position. A SeekableByteChannel
likewise supports truncating the file associated with the channel and querying the file for its size.
The adequacy to move to different points in the file and then read from or write to that location makes random access of a file possible. See Random Access Files for more than data.
In that location are 2 methods for reading and writing channel I/O.
-
newByteChannel(Path, OpenOption...)
-
newByteChannel(Path, Set<? extends OpenOption>, FileAttribute<?>...)
Note: The newByteChannel
methods render an case of a SeekableByteChannel
. With a default file arrangement, you can cast this seekable byte channel to a FileChannel
providing access to more advanced features such mapping a region of the file directly into retention for faster access, locking a region of the file so other processes cannot admission it, or reading and writing bytes from an absolute position without affecting the channel's current position.
Both newByteChannel
methods enable you to specify a list of OpenOption
options. The same open up options used by the newOutputStream
methods are supported, in addition to i more selection: READ
is required considering the SeekableByteChannel
supports both reading and writing.
Specifying READ
opens the channel for reading. Specifying WRITE
or APPEND
opens the channel for writing. If none of these options are specified, so the aqueduct is opened for reading.
The following code snippet reads a file and prints it to standard output:
public static void readFile(Path path) throws IOException { // Files.newByteChannel() defaults to StandardOpenOption.READ attempt (SeekableByteChannel sbc = Files.newByteChannel(path)) { final int BUFFER_CAPACITY = 10; ByteBuffer buf = ByteBuffer.allocate(BUFFER_CAPACITY); // Read the bytes with the proper encoding for this platform. If // y'all skip this step, you might meet strange or illegible // characters. String encoding = System.getProperty("file.encoding"); while (sbc.read(buf) > 0) { buf.flip(); System.out.print(Charset.forName(encoding).decode(buf)); buf.articulate(); } } }
The following example, written for UNIX and other POSIX file systems, creates a log file with a specific set up of file permissions. This code creates a log file or appends to the log file if it already exists. The log file is created with read/write permissions for owner and read only permissions for group.
import static java.nio.file.StandardOpenOption.*; import coffee.nio.*; import java.nio.channels.*; import java.nio.file.*; import java.nio.file.attribute.*; import java.io.*; import java.util.*; public class LogFilePermissionsTest { public static void main(String[] args) { // Create the set of options for appending to the file. Prepare<OpenOption> options = new HashSet<OpenOption>(); options.add(APPEND); options.add together(CREATE); // Create the custom permissions attribute. Set<PosixFilePermission> perms = PosixFilePermissions.fromString("rw-r-----"); FileAttribute<Set up<PosixFilePermission>> attr = PosixFilePermissions.asFileAttribute(perms); // Convert the string to a ByteBuffer. String s = "Hullo Earth! "; byte information[] = s.getBytes(); ByteBuffer bb = ByteBuffer.wrap(information); Path file = Paths.get("./permissions.log"); effort (SeekableByteChannel sbc = Files.newByteChannel(file, options, attr)) { sbc.write(bb); } grab (IOException x) { Arrangement.out.println("Exception thrown: " + x); } } }
Methods for Creating Regular and Temporary Files
Creating Files
You tin create an empty file with an initial ready of attributes by using the createFile(Path, FileAttribute<?>)
method. For example, if, at the time of creation, yous want a file to take a particular fix of file permissions, use the createFile
method to practise and then. If you do not specify any attributes, the file is created with default attributes. If the file already exists, createFile
throws an exception.
In a unmarried atomic operation, the createFile
method checks for the beingness of the file and creates that file with the specified attributes, which makes the process more secure against malicious lawmaking.
The following lawmaking snippet creates a file with default attributes:
Path file = ...; effort { // Create the empty file with default permissions, etc. Files.createFile(file); } catch (FileAlreadyExistsException x) { System.err.format("file named %s" + " already exists%n", file); } take hold of (IOException x) { // Some other sort of failure, such as permissions. Arrangement.err.format("createFile error: %south%north", x); }
POSIX File Permissions has an example that uses createFile(Path, FileAttribute<?>)
to create a file with pre-set permissions.
Yous can as well create a new file by using the newOutputStream
methods, as described in Creating and Writing a File using Stream I/O. If y'all open up a new output stream and close information technology immediately, an empty file is created.
Creating Temporary Files
You can create a temporary file using 1 of the following createTempFile
methods:
-
createTempFile(Path, Cord, String, FileAttribute<?>)
-
createTempFile(String, String, FileAttribute<?>)
The showtime method allows the code to specify a directory for the temporary file and the second method creates a new file in the default temporary-file directory. Both methods permit yous to specify a suffix for the filename and the commencement method allows yous to also specify a prefix. The following lawmaking snippet gives an example of the 2nd method:
effort { Path tempFile = Files.createTempFile(goose egg, ".myapp"); System.out.format("The temporary file" + " has been created: %s%n", tempFile) ; } take hold of (IOException x) { Organization.err.format("IOException: %s%northward", x); }
The issue of running this file would be something similar the following:
The temporary file has been created: /tmp/509668702974537184.myapp
The specific format of the temporary file name is platform specific.
maddoxidentradmus.blogspot.com
Source: https://docs.oracle.com/javase/tutorial/essential/io/file.html
0 Response to "Reading in a From a File Java"
Post a Comment